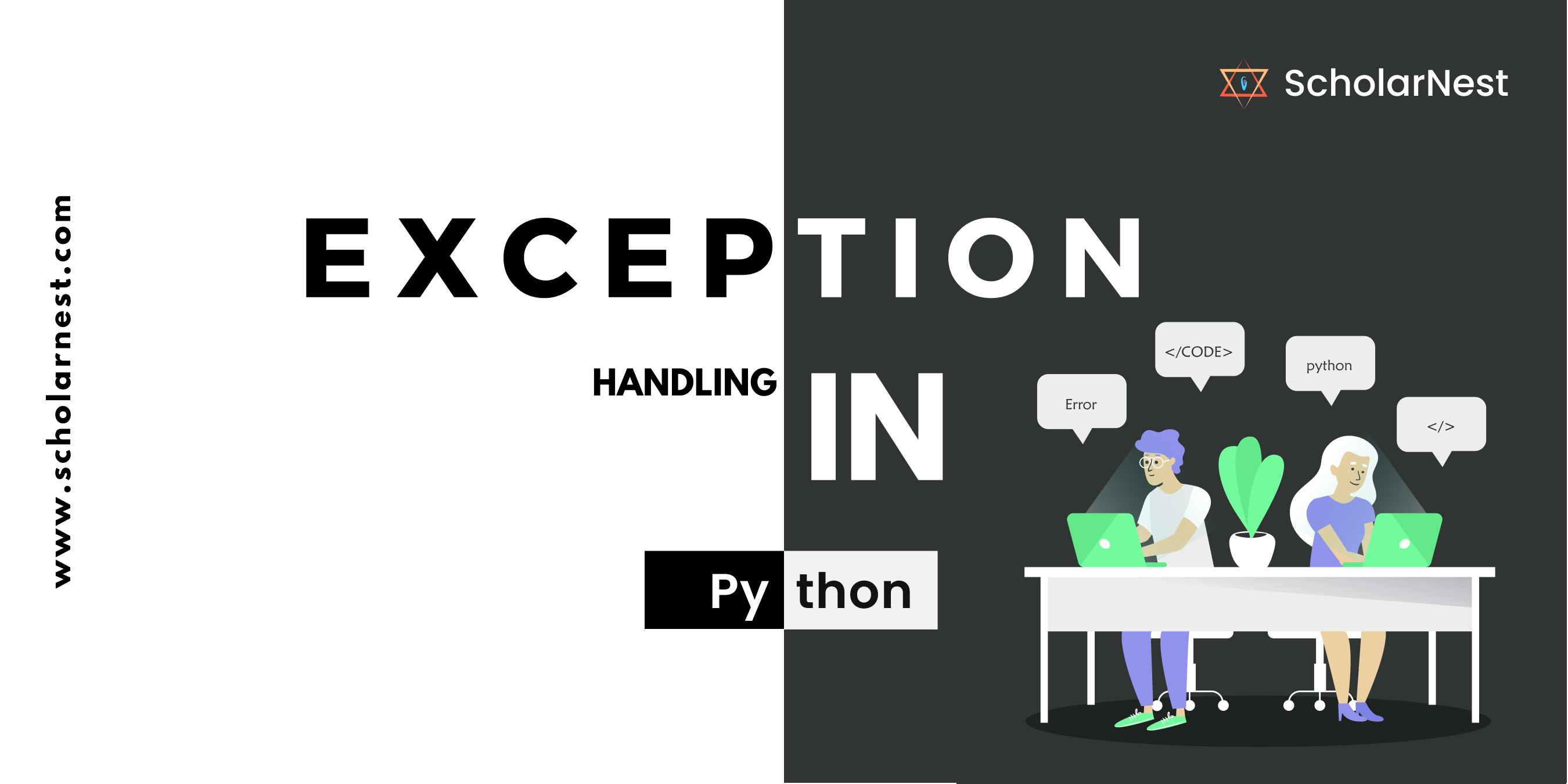
Focus on Exception Handling in Python and it’s types
- ScholarNest
- January 4, 2024
- Programming, Python
Exception Handling in Python
Exception handling is a crucial part of Python programming that helps developers handle unexpected issues in their code smoothly. In this article, we’ll cover different types of exceptions in Python, understand how to use try and except to handle them, explore the try with else clause, see why finally is essential, and learn how to raise exceptions intentionally. We’ll also look at the pros and cons of exception handling in Python to give you a solid grasp of this important concept.
Error vs Exception
A job analysis is a process that aids you to identify and understand the job responsibilities, objectives, tasks, employability skills required and the work environment for a specific position. It is a tool that is used for creating a peerless job description- however there is much more to it. The recruitment process, if carried out smoothly, enhances your growth in the desired position and supports your long term career.
In programming, errors and exceptions are two fundamental concepts that handle issues arising during code execution, but they differ in nature.
-
Errors: Errors are usually problems in the code that are detected before or during compilation. They prevent the program from running, often due to syntax mistakes, missing resources, or incorrect code structure.
-
Exceptions: Exceptions on the other hand, are issues that occur during the execution of a program. They are typically unexpected scenarios that a program isn’t naturally equipped to handle, like attempting to divide by zero or accessing a file that doesn’t exist. While errors are mostly unresolvable by the program itself, exceptions can be caught and handled using specific code structures to maintain normal program flow.
Types of Exceptions in Python
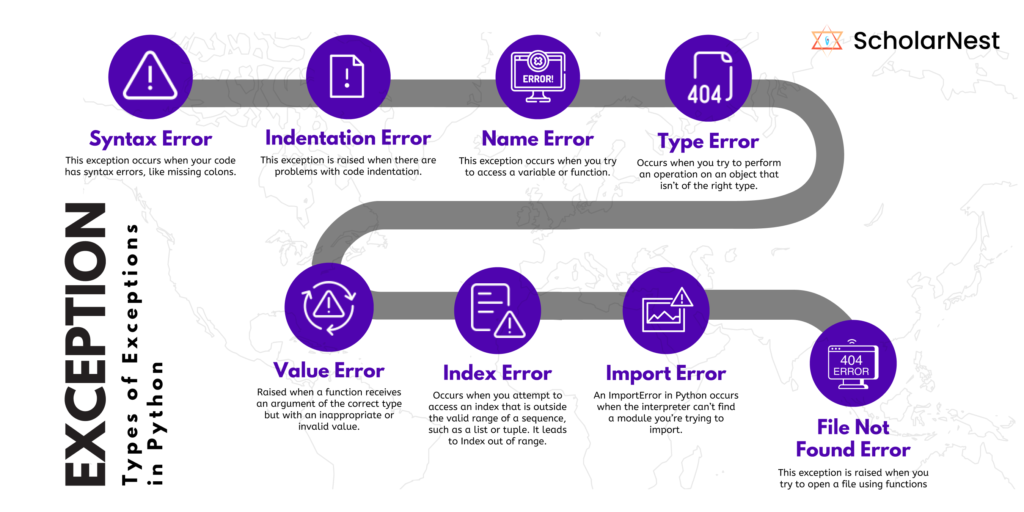
Types of Exceptions in Python
Python offers a wide variety of built-in exceptions, each designed to manage specific error situations. Let’s take a closer look at each type of exception with practical examples:
SyntaxError:
This exception occurs when your code has syntax errors, like missing colons, incorrect indentation, or unbalanced parentheses.
Example:
# SyntaxError: Missing colon
if x
print(“Hello”)
IndentationError:
This exception is raised when there are problems with code indentation, which is crucial in Python for defining block structures.
Example:
# IndentationError: Inconsistent indentation
def my_function():
print(“Indented incorrectly”)
NameError:
This exception occurs when you try to access a variable or function that hasn’t been defined in the current part of your code.
Example:
# NameError:
#name ‘undefined_variable’ is not defined
print(undefined_variable)
TypeError:
Occurs when you try to perform an operation on an object that isn’t of the right type. For example, attempting to combine a string with an integer would trigger this error.
Example:
# TypeError: can only concatenate str (not “int”) to str
result = “Hi! I am a scholar, and I am ” + 24
ValueError:
Raised when a function receives an argument of the correct type but with an inappropriate or invalid value. For example, if you try to convert a non-numeric string into an integer, this exception will be raised.
Example:
# ValueError: invalid literal for int() with base 10: ‘INDIA’
num = int(“INDIA”)
ZeroDivisionError:
This exception occurs when you try to divide a number by zero, which is mathematically undefined.
Example:
# ZeroDivisionError: division by zero
result = 10 / 0
FileNotFoundError:
This exception is raised when you try to open a file using functions like open(), but the file you’re trying to open doesn’t exist.
Example:
# FileNotFoundError: [Errno 2] No such file or directory: ‘main.txt’
file = open(‘main.txt’, ‘r’)
IndexError:
Occurs when you attempt to access an index that is outside the valid range of a sequence, such as a list or tuple. It leads to Index out of range.
Example:
# IndexError: list index out of range
my_list = [1, 2, 3]
value = my_list[10]
KeyError:
This exception is raised when you attempt to access a key in a dictionary that doesn’t exist, typically using square brackets to access a non-existent key.
Example:
# KeyError: ‘nonexistent_key’
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
value = my_dict[‘nonexistent_key’]
ImportError:
An ImportError in Python occurs when the interpreter can’t find a module you’re trying to import.
Example:
import mymodulename
If “mymodulename” doesn’t exist, Python raises an ImportError, indicating it can’t locate this module.
The diagram below illustrates the hierarchy for built-in exceptions, as outlined in the official Python documentation:
BaseException
├── BaseExceptionGroup
├── GeneratorExit
├── KeyboardInterrupt
├── SystemExit
└── Exception
├── ArithmeticError
│ ├── FloatingPointError
│ ├── OverflowError
│ └── ZeroDivisionError
├── AssertionError
├── AttributeError
├── BufferError
├── EOFError
├── ExceptionGroup [BaseExceptionGroup]
├── ImportError
│ └── ModuleNotFoundError
├── LookupError
│ ├── IndexError
│ └── KeyError
├── MemoryError
├── NameError
│ └── UnboundLocalError
├── OSError
│ ├── BlockingIOError
│ ├── ChildProcessError
│ ├── ConnectionError
│ │ ├── BrokenPipeError
│ │ ├── ConnectionAbortedError
│ │ ├── ConnectionRefusedError
│ │ └── ConnectionResetError
│ ├── FileExistsError
│ ├── FileNotFoundError
│ ├── InterruptedError
│ ├── IsADirectoryError
│ ├── NotADirectoryError
│ ├── PermissionError
│ ├── ProcessLookupError
│ └── TimeoutError
├── ReferenceError
├── RuntimeError
│ ├── NotImplementedError
│ └── RecursionError
├── StopAsyncIteration
├── StopIteration
├── SyntaxError
│ └── IndentationError
│ └── TabError
├── SystemError
├── TypeError
├── ValueError
│ └── UnicodeError
│ ├── UnicodeDecodeError
│ ├── UnicodeEncodeError
│ └── UnicodeTranslateError
└── Warning
├── BytesWarning
├── DeprecationWarning
├── EncodingWarning
├── FutureWarning
├── ImportWarning
├── PendingDeprecationWarning
├── ResourceWarning
├── RuntimeWarning
├── SyntaxWarning
├── UnicodeWarning
└── UserWarning
This indicates that IndentationError is a subclass of SyntaxError, and in turn, SyntaxError is a subclass of the Exception class, which is derived from the BaseException.
Try and Except Statement — Catching Exceptions
In Python, when an error or exception occurs, the program typically stops running and shows an error message. To prevent this, Python provides the try and except statements, which are essential for handling exceptions gracefully. Let’s explore with some examples:
try:
# Code with an exception
except ExceptionType:
# Code to handle the exception
- The try block includes code that could cause an error.
- If an exception of the specified ExceptionType is encountered while executing the try block, Python switches the program’s flow to the except block, where you can specify how to manage that particular exception.
For instance:
try:
num = int(input(“Enter a number: “))
result = 10 / num
except ZeroDivisionError:
print(“Error: Cannot divide by zero.”)
In this example, the code within the try block tries to divide 10 by a number entered by the user. If the user inputs 0, which leads to a ZeroDivisionError, the program handles this exception gracefully by displaying an error message.
Try with Else Clause
In Python, you can use the else clause together with the try block. The code within the else block will run only if no exception is raised during the execution of the try block.
try:
# Code with an exception
except ExceptionType:
# Code to handle the exception
else:
# Code that execute if there is no exception
Consider this example:
try:
num = int(input(“Enter a number: “))
except ValueError:
print(“Invalid input. Please enter a valid number.”)
else:
result = 10 / num
print(f”Result: {result}”)
In this scenario, if the user inputs a valid value (which means a non-zero number), the code inside the else block will execute. It calculates and displays the result of the division. However, if the user enters non-numeric input, the except block gracefully handles the ValueError.
Using Finally Block for exception handling in Python
The finally block is a critical part of exception handling in Python. It allows you to specify code that will run no matter what, whether an exception is raised or not. This is often used for essential cleanup operations, like closing files or releasing resources, that need to be performed regardless of how the program’s execution takes place.
try:
# Code with an exception
except ExceptionType:
# Code to handle the exception
finally:
# Code that always executes, such as cleanup operations
Here’s a practical example:
try:
file = open(“example.txt”, “r”)
# Perform operations on the file
except FileNotFoundError:
print(“File not found.”)
finally:
file.close() # Always close the file, whether an exception occurred or not
In this example, the try block is responsible for trying to open a file for reading. If the file doesn’t exist, resulting in a FileNotFoundError, the except block takes care of handling this particular exception. Importantly, the finally block will execute without fail, whether an exception occurs or not, ensuring that the file is closed properly. This guarantees that any open resources are managed correctly.
Raising Exceptions
In Python, you have the ability to raise exceptions intentionally by using the raise keyword. This capability becomes particularly valuable when you want to create custom exceptions tailored to your specific requirements or when you need to handle particular cases in your code with precision and control.
def divide(x,y):
if y == 0:
raise ZeroDivisionError(“Division by zero is not allowed”)
return x / y
try:
result = divide(10, 0)
except ZeroDivisionError as e:
print(e)
In the given example, the divide function intentionally raises a ZeroDivisionError if the denominator (y) is equal to zero. When the function is called with divide(10, 0), this specific exception is deliberately triggered, and the except block is responsible for handling it by displaying the associated error message. This demonstrates how you can use the raise keyword to control and manage exceptions in your code.
Advantages and Disadvantages of Exception Handling
Advantages
Exceptional management has several major benefits:
Improved Program Robustness:
Exception handling is a powerful tool because it makes your program more robust. It lets you deal with errors gracefully, so your program doesn’t crash abruptly when unexpected issues arise. Instead of stopping everything, exception handling helps you manage errors in a controlled way. This means your application can keep running, offering users a smoother experience even when faced with unexpected challenges.
Separation of Concerns:
Another great thing about using exception handling is that it helps keep your code neat and organized. It separates error-handling from the main part of your code, making it easier to understand and maintain. This way, you can focus on your code’s core functions in one place, while handling errors separately in a way that keeps everything organized and easy to follow.
Debugging Aid:
Exception messages are like helpful clues when you’re debugging your code. They give you valuable information about the errors, providing context and details about what went wrong during the program’s execution. These messages make it easier to pinpoint and fix issues, saving you time and effort in the debugging process.
Custom Error Handling:
Python allows you to create custom exceptions, tailoring error handling to your application’s unique needs. This precision enhances error management, making it easier to identify and address issues effectively.
Disadvantages
While exception handling is a powerful tool, it does have some drawbacks:
Performance Overhead:
One disadvantage of exception handling is that it can introduce a performance overhead when compared to using simple conditional checks. This overhead may impact the speed of highly optimized code, making it slightly less efficient.
Complexity:
Overusing or misusing exceptions can make code more complex and challenging to understand. This can lead to maintainability issues, as excessively intricate exception handling can obscure the main logic of your code, making it harder to maintain and troubleshoot.
Hidden Errors:
Another disadvantage of poorly handled exceptions is that they can mask underlying issues in your code. When exceptions are not handled correctly, debugging becomes more challenging and time-consuming because the true cause of an error might be hidden by improper exception handling, leading to confusion during the debugging process.
Overhead in Small-Scale Programs:
In very basic programs or scripts, using exception handling may not be warranted, as it can introduce unnecessary complexity and overhead. In such cases, simple conditional checks and error handling may be a more straightforward and efficient approach.
Conclusion
Proficiency in exception handling in Python is important for writing reliable code. It helps you deal with unexpected issues in a smooth way and makes your code easier to maintain and read. However, it’s important to use exception handling wisely, considering both its benefits and drawbacks. By doing this, you can create Python programs that work well and remain stable even when faced with unexpected problems. Exception handling is a valuable skill for Python programmers, and learning how and when to use it will greatly improve your coding abilities.