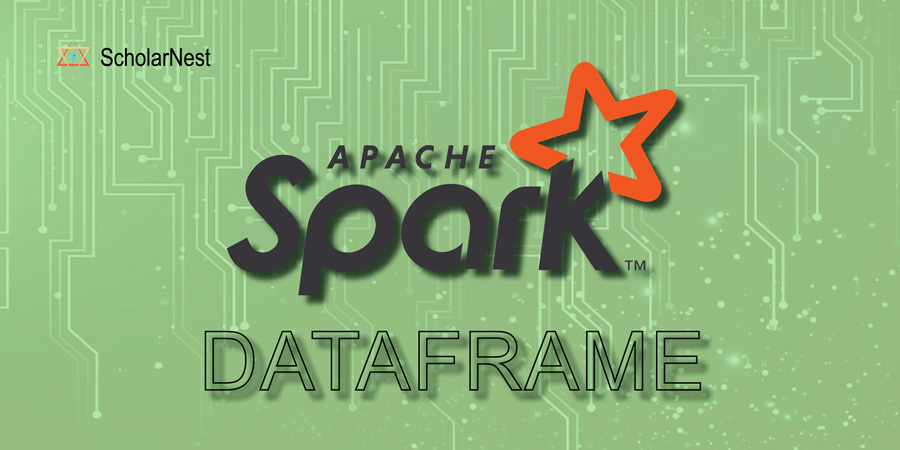
Unique and New Ways to Create Spark Dataframe | Scholarnest
- ScholarNest
- March 19, 2023
- Apache Spark
Spark Dataframe
Apache Spark is a powerful open-source distributed computing framework that provides efficient and scalable processing of large datasets. One of the key features of Spark is its ability to handle structured data using a powerful data abstraction called Spark Dataframe. Spark Dataframe are similar to tables in a relational database, and they provide a high-level API for processing structured data.
Importance of creating Spark Dataframe
Creating Spark Dataframe is an essential step in most data processing and analysis tasks using Spark. Spark Dataframe can be used to manipulate, transform, and aggregate data efficiently using various Spark transformations and actions. Moreover, Spark Dataframe can be integrated with various data sources such as CSV, JSON, and Parquet files, SQL databases, Hive tables, and many more.
Overview of different ways to create Spark Dataframe
There are several ways to create Spark Dataframe in Spark. Some of the most used methods include the following.
-
- Creating Spark Dataframe programmatically using a list or dictionary
-
- Creating Spark Dataframe from external data sources
-
- Creating Spark Dataframe using SQL queries
-
- Creating Spark Dataframe using SparkSession
-
- Creating Spark Dataframe using Hive tables
In this blog post, we will explore each of these methods in detail and discuss their advantages and disadvantages.
Programmatically creating Spark Dataframe
Programmatically creating Spark Dataframe is a common way to create a Spark Dataframe in PySpark. In this approach, we write code to create a Spark Dataframe using the PySpark API.
To create a Spark Dataframe programmatically, we can use the createDataFrame() method of the SparkSession object. The createDataFrame() method takes two arguments – the data and the schema. The data can be in different formats, such as a list of tuples, a list of dictionaries, or an RDD. The schema is a StructType object that defines the structure of the data.
Here is an example of creating a Spark Dataframe using a list of tuples in PySpark:
from pyspark.sql import SparkSession from pyspark.sql.types import StructType, StructField, IntegerType, StringType # Create a SparkSession object spark = SparkSession.builder \ .appName("Programmatically creating Spark Dataframe") \ .getOrCreate() # Define the schema for the data schema = StructType([ StructField("name", StringType(), True), StructField("age", IntegerType(), True), StructField("city", StringType(), True)]) # Create the data data = [("Alice", 25, "New York"), ("Bob", 30, "San Francisco"), ("Charlie", 35, "Seattle")] # Create the Spark Dataframe df = spark.createDataFrame(data, schema) # Show the contents of the Spark Dataframe df.show()
In the above example, we first create a SparkSession object. Then we define the schema for the data using the StructType object. After that, we create the data as a list of tuples. Finally, we create the Spark Dataframe using the createDataFrame() method and pass the data and schema as arguments. We can then display the contents of the Spark Dataframe using the show() method.
We can also create a Spark Dataframe using a list of dictionaries. Here is an example:
from pyspark.sql import SparkSession from pyspark.sql.types import StructType, StructField, IntegerType, StringType # Create a SparkSession object spark = SparkSession.builder \ .appName("Programmatically creating Spark Dataframe") \ .getOrCreate() # Define the schema for the data schema = StructType([ StructField("name", StringType(), True), StructField("age", IntegerType(), True), StructField("city", StringType(), True)]) # Create the data data = [{"name": "Alice", "age": 25, "city": "New York"}, {"name": "Bob", "age": 30, "city": "San Francisco"}, {"name": "Charlie", "age": 35, "city": "Seattle"}] # Create the Spark Dataframe df = spark.createDataFrame(data, schema) # Show the contents of the Spark Dataframe df.show()
In this example, we define the schema and create the data as a list of dictionaries. Then, we create the Spark Dataframe using the createDataFrame() method and pass the data and schema as arguments.
Programmatically creating Spark Dataframe is a powerful way to create Dataframe in PySpark as it allows us to create Dataframe from a sample data set.
Creating Spark Dataframe from external data sources
Apart from programmatically creating Apache Spark Dataframe, Spark also allows creating Dataframe from external data sources. Some of the commonly used data sources to create Dataframe in Apache Spark include CSV, JSON, Parquet, and JDBC.
Creating Dataframe from CSV and JSON files
Creating Dataframe from CSV and JSON files is a popular way of creating a Dataframe in Apache Spark. The CSV and JSON files can be stored locally or on a distributed file system like HDFS or S3. The following code shows how to create a Dataframe from a CSV file using PySpark:
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName('CSV to Dataframe') \ .getOrCreate() df = spark.read \ .csv('path/to/file.csv', header=True, inferSchema=True) df.show()
Here, we are using the read.csv() function to read the CSV file and create a Dataframe. The header parameter is set to True, indicating that the first row in the CSV file contains the header. The inferSchema parameter is set to True, which infers the schema of the Dataframe based on the data in the CSV file.
The following code shows how to create a Dataframe from a JSON file using PySpark:
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName('JSON to Dataframe') \ .getOrCreate() df = spark.read.json('path/to/file.json') df.show()
Here, we are using the read.json() function to read the JSON file and create a Dataframe.
Creating Apache Spark Dataframe from Parquet files
Parquet is a columnar storage format that is highly optimized for large-scale data processing. Creating Dataframe from Parquet files can significantly improve the performance of Spark applications. The following code shows how to create a Dataframe from a Parquet file using PySpark:
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName('Parquet to Dataframe') \ .getOrCreate() df = spark.read.parquet('path/to/file.parquet') df.show()
Here, we are using the read.parquet() function to read the Parquet file and create a dataframe.
Creating Dataframe from JDBC
Spark also allows creating Dataframe from external databases using JDBC. The following code shows how to create a dataframe from a MySQL database table using PySpark:
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName('JDBC to Dataframe') \ .getOrCreate() jdbc_url = 'jdbc:mysql://<host>:<port>/<database>' table_name = '<table_name>' properties = {'user': '<username>', 'password': '<password>'} df = spark.read \ .jdbc(url=jdbc_url, table=table_name, properties=properties) df.show()
Here, we are using the read.jdbc() function to read the MySQL database table and create a dataframe. The jdbc_url parameter contains the connection URL of the MySQL database. The table_name parameter contains the name of the table to read. The properties parameter contains the username and password to connect to the MySQL database.
In conclusion, Spark provides different ways to create Dataframe, including programmatically creating Dataframe and creating Dataframe from CSV, JSON, Parquet, and JDBC sources. These Dataframe can be used to perform various data processing operations using Spark SQL and Dataframe API.
Creating Spark Dataframe using SQL queries
Another way to create Spark Dataframe is by using SQL queries.Apache Spark allows us to create Dataframe from various sources such as JSON, CSV, Parquet, Avro, ORC, and JDBC data sources. We can register a table from the external data source and run SQL queries to create a Dataframe.
Here is an example of how to create a Dataframe using SQL queries in PySpark:
from pyspark.sql import SparkSession # create a SparkSession spark = SparkSession.builder \ .appName("CreateDF") \ .getOrCreate() # create a temporary table from a CSV file df = spark.read \ .format("csv") \ .option("header", "true") \ .load("sample.csv") df.createOrReplaceTempView("temp_table") # create a DataFrame using a SQL query new_df = spark.sql("SELECT * FROM temp_table WHERE age >= 18") # display the new DataFrame new_df.show()
In the code above, we first create a SparkSession. We then read in a CSV file and create a temporary table from it using the createOrReplaceTempView() function. We can then run SQL queries on the temporary table using the spark.sql() function. In this example, we select all the rows where the age is greater than or equal to 18 and create a new Dataframe called new_df. We can then display the contents of the new Dataframe using the show() function.
Creating Dataframe using SQL queries can be especially useful when we need to join multiple data sources together or when we need to filter or aggregate large amounts of data. Additionally, by using SQL queries, we can take advantage of Spark’s optimized query engine to improve the performance of our data processing tasks.
Creating Spark Dataframe using SparkSession
Apart from using the above three methods, we can also create Spark Dataframe using SparkSession. SparkSession is a unified entry point of a Spark application, and it provides a way to interact with various Spark functionality like Spark SQL, Spark Streaming, and Machine Learning.
SparkSession provides the createDataFrame() method to create a DataFrame from a list, pandas DataFrame, or RDD. We can also specify the schema of the DataFrame while creating it. Let’s look at some PySpark examples to understand this method.
Example 1: Creating a DataFrame from a list
from pyspark.sql import SparkSession # Creating SparkSession spark = SparkSession.builder \ .appName("Spark Dataframe") \ .getOrCreate() # Creating a list of tuples data = [('Alice', 25), ('Bob', 30), ('Charlie', 35)] # Creating a DataFrame from a list of tuples df = spark.createDataFrame(data, ['Name', 'Age']) # Displaying the DataFrame df.show()
Example 2: Creating a DataFrame from a pandas DataFrame
from pyspark.sql import SparkSession import pandas as pd # Creating SparkSession spark = SparkSession.builder \ .appName("Spark Dataframe") \ .getOrCreate() # Creating a pandas DataFrame data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]} pandas_df = pd.DataFrame(data) # Creating a DataFrame from pandas DataFrame df = spark.createDataFrame(pandas_df) # Displaying the DataFrame df.show()
Example 3: Creating a Dataframe from an RDD
from pyspark.sql import SparkSession from pyspark.sql.types import StructType, StructField, StringType, IntegerType # Creating SparkSession spark = SparkSession.builder \ .appName("Spark Dataframe") \ .getOrCreate() # Creating an RDD rdd = spark.sparkContext.parallelize([(1, 'Alice'), (2, 'Bob'), (3, 'Charlie')]) # Creating schema for the DataFrame schema = StructType([ StructField("Id", IntegerType(), True), StructField("Name", StringType(), True)]) # Creating a DataFrame from RDD and schema df = spark.createDataFrame(rdd, schema) # Displaying the DataFrame df.show()
In the above examples, we have seen how to create a Spark DataFrame using SparkSession. We can create a DataFrame from a list, pandas DataFrame, or RDD. SparkSession provides us the flexibility to create Dataframe in multiple ways.
Creating Spark Dataframe using Hive tables
Apache Hive is a popular data warehouse tool that enables data summarization, querying and analysis of large datasets stored in Hadoop. It uses a SQL-like language called HiveQL, which is like SQL, to process and analyze data. One of the advantages of Hive is that it allows users to define tables that are stored in Hadoop Distributed File System (HDFS), making it easier to query large datasets.
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName("HiveTableDemo") \ .enableHiveSupport() .getOrCreate() # enableHiveSupport() enables the use of HiveContext # getOrCreate() gets the existing SparkSession or creates a new one if none exists
Once you have created the SparkSession object, you can use it to create a DataFrame from a Hive table.
# creating dataframe from hive table using table name df_hive = spark.table("database_name.table_name") # creating dataframe from hive table using sql query df_hive = spark.sql("SELECT * FROM database_name.table_name")
The above code creates a Dataframe called df_hive by querying the Hive table named table_name in the database named database_name. Alternatively, you can also create a Spark Dataframe from a Hive table using an SQL query.
# displaying the contents of the Dataframe df_hive.show()
You can now perform various transformations and actions on the df_hive DataFrame, just like any other DataFrame created using other methods.
# performing transformations and actions df_hive.select("col1", "col2").filter("col3 > 100").show()
In the above code, we select the columns col1 and col2 from the df_hive DataFrame and apply a filter on col3 where its value is greater than 100.
In summary, creating Spark Dataframe from Hive tables is a simple process in PySpark. All you need is a SparkSession object and knowledge of the table or SQL query that you want to use. Once you have created the DataFrame, you can perform various transformations and actions on it to analyze and process the data.
Conclusion
In conclusion, there are multiple ways to create Spark Dataframe in PySpark. Depending on the use case and data source, one can choose the most appropriate method. Programmatically creating Dataframe offers a lot of flexibility and control over the schema and data, whereas creating Dataframe from external data sources or using SQL queries can save time and effort. SparkSession and Hive tables also provide convenient ways to create Dataframe.
It is essential to choose the right method for creating a Dataframe to optimize the performance and scalability of the Spark application. Moreover, it is equally important to understand the limitations and trade-offs of each method.
In summary, Spark Dataframe is a powerful and flexible way to work with structured data in PySpark. With multiple options to create Dataframe, developers can choose the most efficient method that suits their needs.
Follow Us For more details check our videos and visit our website.